TUTORIAL 4
INPUT AND OUTPUT
Outputting Terms
The write/1 predicate takes a single argument, which must be a valid Prolog term. Evaluating the predicate causes the term to be written to the current output stream, which by default is the user's screen. Evaluating a nl goal causes a new line to be output to the current output stream.
Examples
?- write(26),nl.
26
yes
?- write('a string of characters'),nl.
a string of characters
yes
?- write([a,b,c,d,[x,y,z]]),nl.
[a,b,c,d,[x,y,z]]
yes
?- write(mypred(a,b,c)),nl.
mypred(a,b,c)
yes
?- write('Example of use of nl'),nl,nl,write('end of example'),nl.
Example of use of nl
end of example
yes
Note that atoms that have to be quoted on input (e.g. 'Paul', 'hello world') are not quoted when output using write. If it is important to output the quotes, the writeq/1 predicate can be used. It is identical to write/1 except that atoms that need quotes for input are output between quotes (other atoms are not).
?- writeq('a string of characters'),nl.
'a string of characters'
yes
?-writeq(dog),nl.
dog
yes
?- writeq('dog'),nl.
dog
yes
Inputting Terms
The built-in predicate read/1 is provided to input terms. It takes a single argument, which must be a variable. Evaluating it causes the next term to be read from the current input stream, which by default is the user's keyboard. In the input stream, the term must be followed by a dot ('.') and at least onewhite space character, such as space or newline. The dot and white space characters are read in but are not considered part of the term. Note that for input from the keyboard (only) a prompt character such as a colon will usually be displayed to indicate that user input is required. It may be necessaryto press the 'return' key before Prolog will accept the input. Both of these do not apply to input from files.When a read goal is evaluated, the input term is unified with the argument variable. If the variable is unbound (which is usually the case) it is bound to the input value.
?- read(X).
: jim.
X = jim
?- read(X).
: 26.
X = 26
?- read(X).
: mypred(a,b,c).
X = mypred(a,b,c)
?- read(Z).
: [a,b,mypred(p,q,r),[z,y,x]].
Z = [a,b,mypred(p,q,r),[z,y,x]]
?- read(Y).
: 'a string of characters'.
Y = 'a string of characters'
If the argument variable is already bound (which for most users is far more likely to occur by mistake than by design), the goal succeeds if and only if the input term is identical to the previously bound value.
?- X=fred,read(X).
: jim.
no
?- X=fred,read(X).
: fred.
X = fred
Input and Output Using CharactersAlthough input and output of terms is straightforward, the use of quotes and full stops can be cumbersome and is not always suitable. For example, it would be tedious to define a predicate (using read) which would read a series of characters from the keyboard and count the number of vowels. A much better approach for problems of this kind is to input a character at a time. To do this it is first necessary to know about the ASCII value of a character.All printing characters and many non-printing characters (such as space and tab) have a corresponding ASCII (American Standard Code for Information Interchange) value, which is an integer from 0 to 255.
Outputting Characters
Characters are output using the built-in predicate put/1. The predicate takes a single argument, which must be a number from 0 to 255 or an expression that evaluates to an integer in that range.Evaluating a put goal causes a single character to be output to the current output stream. This is the character corresponding to the numerical value (ASCII value) of its argument, for example
?- put(97),nl.
a
yes
?- put(122),nl.
z
yes
?- put(64),nl.
@
yes
Inputting Characters
Two built-in predicates are provided to input a single character: get0/1 and get/1.The get0 predicate takes a single argument, which must be a variable. Evaluating aget0 goal causes a character to be read from the current input stream. The variableis then unified with the ASCII value of this character.Note that for input from the keyboard (only) a prompt character such as a colonwill usually be displayed to indicate that user input is required. It may be necessaryto press the 'return' key before Prolog will accept the input. Both of these alsoapply to the get predicate described below but do not apply to input from files.Assuming the argument variable is unbound (which will usually be the case), itis bound to the ASCII value of the input character.
?- get0(N).
: a
N = 97
?- get0(N).
: Z
N = 90
74 Logic Programming With Prolog
?- get0(M).
: )M = 41
If the argument variable is already bound, the goal succeeds if and only if it has a numerical value that is equal to the ASCII value of the input character.
?- get0(X).
: a
X = 97
?- M is 41,get0(M).
: )
M = 41
?- M=dog,get0(M).
: )
no
?- M=41.001,get0(M).
: )
no
The get predicate takes a single argument, which must be a variable. Evaluatinga get goal causes the next non-white-space character (i.e. character with an ASCIIvalue less than or equal to 32) to be read from the current input stream. Thevariable is then unified with the ASCII value of this character in the same way asfor get0.
?- get(X).
: Z
X = 90
?- get(M).
: Z
M = 90
Rabu, 25 November 2009
Selasa, 24 November 2009
Expert System Design
Our simple design of expert system is:
"What is your suitable major?"
Background
We often find some student that feel confuse to decide what major they will take for their next study. Sometimes they fell hesitate to ask their teacher, or some other case just like leak of information, and difficult to get information about Universities. So because of that we want to help them
Explanation
This expert system is made for senior high school student to determine what kind of major that suitable for them. We make a question about their interest, favourite subject, their dream/idea. etc. And then, after they answer the multiple choice, we show the suitable major based o our characteristic that we have determine it before.
Benefit
The benefit is to help senior high school student in order not to take wrong major. We don't want they take wrong major that not suitable for their dream. They can explore their ability if they like the major itself.
"What is your suitable major?"
Background
We often find some student that feel confuse to decide what major they will take for their next study. Sometimes they fell hesitate to ask their teacher, or some other case just like leak of information, and difficult to get information about Universities. So because of that we want to help them
Explanation
This expert system is made for senior high school student to determine what kind of major that suitable for them. We make a question about their interest, favourite subject, their dream/idea. etc. And then, after they answer the multiple choice, we show the suitable major based o our characteristic that we have determine it before.
Benefit
The benefit is to help senior high school student in order not to take wrong major. We don't want they take wrong major that not suitable for their dream. They can explore their ability if they like the major itself.
Kamis, 12 November 2009
Blog kami
1. Irine Dwi Kenestie http://irindisini.blogspot.com/
2. Rizqi Prifsanti http://kucinglewat.blogspot.com/
3. Bunga Fadhila http://bungaitusaya.blogspot.com/
2. Rizqi Prifsanti http://kucinglewat.blogspot.com/
3. Bunga Fadhila http://bungaitusaya.blogspot.com/
Summary : Operator and Arithmetic in Prolog
1. Operator
Binary predicate (predikat dengan dua argumen) dapat diubah menjadi bentuk infix operator. Contoh :
Standar : makan (kucing,tikus)
infix operator : kucing makan tikus
Unary predikat (predikat dengan satu argumen) dapat diubah menjadi bentuk prefix operator atau postfix operator. Contoh :
Standar : lucu (anjing)
prefix operator : lucu anjing
postfix operator: anjing lucu
2. Aritmatika
Dengan Prolog kita dapat melakukan penghitungan aritmatika.
a. Operator Aritmatika
X+Y (penjumlahan)
X-Y (pengurangan)
X*Y (perkalian)
X/Y (pembagian)
X//Y the ‘integer quotient’ of X and Y (the result is truncated to the nearest integer between it and zero)
X^Y (pangkat)
-X (negatif)
abs(X) (nilai absolut)
sin(X) (sinus)
cos(X) (cosinus)
max(X,Y)(nilai terbesar)
sqrt(X) (akar)
b. Pengutamaan Operator Dalam Ekspresi Aritmatika
Prolog menggunakan algoritma aljabar biasa dalam pengopersian aritmatika. Contohnya A+B*C-D. Di dalam ajabar C dan D dikalikan lebih dahulu lalu ditambah dengan A lalu dikurangi dengan D. DI prolog juga demikian. Untuk pengecualian, kita tinggal menggunakan kurung. Contoh : (A+B)*(C+D).
c. Operator Relasi
Operator seperti =, !=, >,>=, <, =<, dapat digunakan di Prolog.
3. Operator Pembanding
Berikut merupakan daftar dari equality operators yang digunakan dalam prolog beserta fungsi dari masing-masing operator.
Arithmetic Expression Equality =:=
Arithmetic Expression Inequality =\=
Terms Identical ==
Terms Not Identical \==
Terms Identical With Unification =
Non-Unification Between Two Terms \=
4. Operator Logika
a. Operator Not
Operator not dapat ditempatkan sebelum predikat untuk memberikan negasi. Predikat yang dinegasikan bernilai benar jika predikat yang asli salah dan bernilai salah jika predikat yang asli benar. Contoh penggunaan operator not :
dog(fido).
?- not dog(fido).
no
?- dog(fred).
no
?- not dog(fred).
yes
b. Operator Disjungsi
Operator disjungsi (;) digunakan sebagai operator ‘atau’. Contoh :
?- 6<3;7 is 5+2.
yes
?- 6*6=:=36;10=8+3.
yes
Binary predicate (predikat dengan dua argumen) dapat diubah menjadi bentuk infix operator. Contoh :
Standar : makan (kucing,tikus)
infix operator : kucing makan tikus
Unary predikat (predikat dengan satu argumen) dapat diubah menjadi bentuk prefix operator atau postfix operator. Contoh :
Standar : lucu (anjing)
prefix operator : lucu anjing
postfix operator: anjing lucu
2. Aritmatika
Dengan Prolog kita dapat melakukan penghitungan aritmatika.
a. Operator Aritmatika
X+Y (penjumlahan)
X-Y (pengurangan)
X*Y (perkalian)
X/Y (pembagian)
X//Y the ‘integer quotient’ of X and Y (the result is truncated to the nearest integer between it and zero)
X^Y (pangkat)
-X (negatif)
abs(X) (nilai absolut)
sin(X) (sinus)
cos(X) (cosinus)
max(X,Y)(nilai terbesar)
sqrt(X) (akar)
b. Pengutamaan Operator Dalam Ekspresi Aritmatika
Prolog menggunakan algoritma aljabar biasa dalam pengopersian aritmatika. Contohnya A+B*C-D. Di dalam ajabar C dan D dikalikan lebih dahulu lalu ditambah dengan A lalu dikurangi dengan D. DI prolog juga demikian. Untuk pengecualian, kita tinggal menggunakan kurung. Contoh : (A+B)*(C+D).
c. Operator Relasi
Operator seperti =, !=, >,>=, <, =<, dapat digunakan di Prolog.
3. Operator Pembanding
Berikut merupakan daftar dari equality operators yang digunakan dalam prolog beserta fungsi dari masing-masing operator.
Arithmetic Expression Equality =:=
Arithmetic Expression Inequality =\=
Terms Identical ==
Terms Not Identical \==
Terms Identical With Unification =
Non-Unification Between Two Terms \=
4. Operator Logika
a. Operator Not
Operator not dapat ditempatkan sebelum predikat untuk memberikan negasi. Predikat yang dinegasikan bernilai benar jika predikat yang asli salah dan bernilai salah jika predikat yang asli benar. Contoh penggunaan operator not :
dog(fido).
?- not dog(fido).
no
?- dog(fred).
no
?- not dog(fred).
yes
b. Operator Disjungsi
Operator disjungsi (;) digunakan sebagai operator ‘atau’. Contoh :
?- 6<3;7 is 5+2.
yes
?- 6*6=:=36;10=8+3.
yes
Fact, Rules, Predicate, and Variable
(1) Type the following program into a file and load it into Prolog.
/* Animals Database */animal(mammal,tiger,carnivore,stripes).animal(mammal,hyena,carnivore,ugly).animal(mammal,lion,carnivore,mane).animal(mammal,zebra,herbivore,stripes).animal(bird,eagle,carnivore,large).animal(bird,sparrow,scavenger,small).animal(reptile,snake,carnivore,long).animal(reptile,lizard,scavenger,small).
Devise and test goals to find (a) all the mammals, (b) all the carnivores that are mammals, (c) all the mammals with stripes, (d) whether there is a reptile that has a mane.
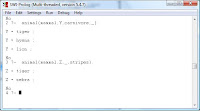
/* Animals Database */animal(mammal,tiger,carnivore,stripes).animal(mammal,hyena,carnivore,ugly).animal(mammal,lion,carnivore,mane).animal(mammal,zebra,herbivore,stripes).animal(bird,eagle,carnivore,large).animal(bird,sparrow,scavenger,small).animal(reptile,snake,carnivore,long).animal(reptile,lizard,scavenger,small).
Devise and test goals to find (a) all the mammals, (b) all the carnivores that are mammals, (c) all the mammals with stripes, (d) whether there is a reptile that has a mane.
1. Pertama-tama buatlah new file di SWIProlog. Buat database seperti ini
2. untuk mengerjakan soal (a) all the mammals ketik ?- animal(mammal,X,_,_). kemudian tekan enter dan ; untuk hasil selanjutnya
3. untuk mengerjakan soal (b) all the carnivores that are mammals, ketik ?- animal(mammal,Y,carnivore,_). kemudian tekan enter dan ; untuk hasil selanjutnya
4. untuk mengerjakan soal (c) all the mammals with stripes, ketik ?- animal(mammal,Z,_,stripes).kemudian tekan enter dan ; untuk hasil selanjutnya
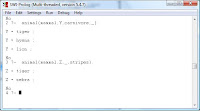
5. untuk mengerjakan soal (d) whether there is a reptile that has a mane, ketik ?- animal(reptile,R,_,mane). kemudian tekan enter dan ; untuk hasil selanjutnya . Jawabannya adalah No, karena tidak ada reptile yang mempunyai mane di dalam database.
(2) Type the following program into a file
/* Dating Agency Database */person(bill,male).person(george,male).person(alfred,male).person(carol,female).person(margaret,female).person(jane,female).
Extend the program with a rule that defines a predicate couple with two arguments, the first being the name of a man and the second the name of a woman.Load your revised program into Prolog and test it.
/* Dating Agency Database */person(bill,male).person(george,male).person(alfred,male).person(carol,female).person(margaret,female).person(jane,female).
Extend the program with a rule that defines a predicate couple with two arguments, the first being the name of a man and the second the name of a woman.Load your revised program into Prolog and test it.
1. Buat database seperti berikut ini
2. Lalu masukkan perintah seperti berikut
?- pasangan(X,Y), lalu tekan enter dan ; untuk hasil selanjutnya
Langganan:
Postingan (Atom)